Hello,
this guide is dedicated to how to create a bot using Aoi.js!
This guide is intended to serve as a alternative to the getting started page of the original docs.
Following words would refer to:
BOT refers to the bot you will be using to code.
HOST or PTERO HOST or PETRO refers to a host using pterodactyl panel.
SERVER refers to the space in a ptero host where you manages or code your bot.
DISCORD DEVELOPER PORTAL or DEV PORTAL refers to the official discord site/portal where you manage your bots. From the portal you get your bot token.
What is Aoi.js?
Aoi.js is a string-based package built using Nodejs with simplified and ready-to-use functions for Discord bot developers. It serves as a wrapper for Discord.js. It aims to be the easiest package to learn and provides swift and flexible functionality using functions.
Features of Aoi.js?
640+ Pre-built Functions: aoi.js comes fully-packaged with over 640 pre-built functions that empower you to create dynamic and interactive Discord bots with much ease.
Built-in Custom Local Database: With aoi.js, you get a powerful custom local database right into it.
Extensions for Added Functionality: Enhance your botās capabilities with aoi.js extensions like @akarui/aoi.music, @akarui/aoi.invite and aoi.panel. These extensions make it simple to add music playback, invite functions, interactive panels, and more to your bot. We also got up extensions created by very users of aoi.js like aoi.canvas or aoi.jikan, which can be used to create canvases.
Easy-to-Use and Beginner Friendly: aoi.js boasts a user-friendly syntax that is perfect for beginners. The simple $
prefix makes it easy to write commands and get your bot up and running quickly.
Custom Functions: Can't find a function that you know and know how to use Discord.js? Create your own Custom Function. Aoi.js comes with the ability to make your own custom function.
So let' get started on how to create.
Let's discuss the steps to create a working bot using aoi.js.
We will be here using a Ptero Host.
Just so you know, we require NodeJS v20 or above to use aoi.js. You can always check this by going to Your Server > Startup > Docker Image, you can check there what your NodeJS version is. If it is lower than v20, you may change it if your host allows or else you will need to use another host.
STARTUP - Mobile
STARTUP - Desktop
DOCKER IMAGE- Mobile
DOCKER IMAGE- Desktop
Step 1. Create a bot in Discord Developer Portal (I don't have a guide on it so for now google it)
Step 2. Create a server with the egg/language NodeJS
or in some host it could be discordJS
, according to the instructions given by the host operators. Can be different depending upon how they let you create a server.
Step 3. As Ptero Host doesn't allow users to type in the terminal so we have to use other methods to install aoi.js.
First Method, Create a file called package.json
and put the following code.
{
"dependencies": {
"aoi.js": "^6.7.1"
}
}
Package.json - Mobile
Package.json - Desktop
This will now allow you to install aoi.js in your server.
Second Method, Go to Your Server -> Startup -> Additional Node Packages, type there aoi.js
. It will install it then.
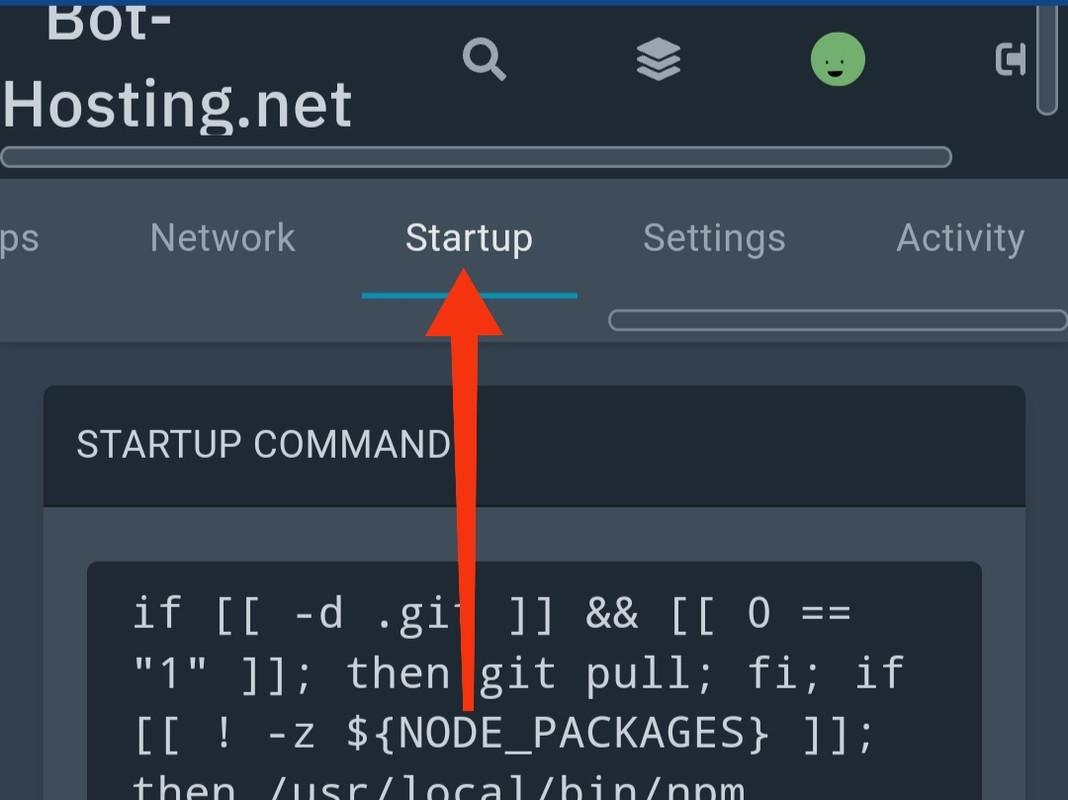
STARTUP - Mobile
STARTUP - Desktop
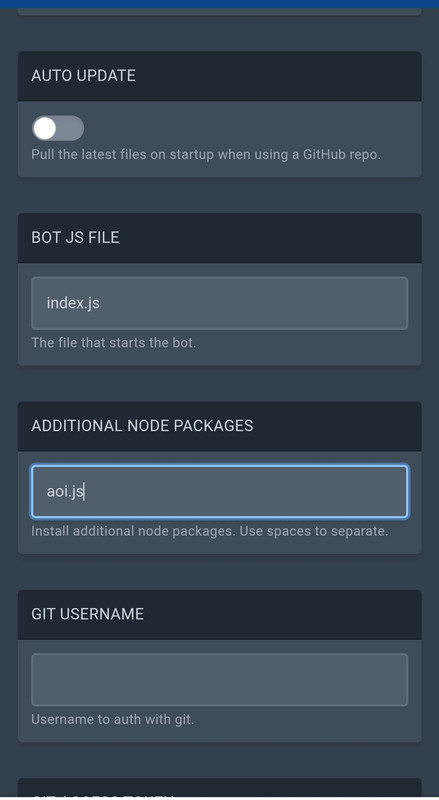
ADDITIONAL NODE PACKAGES - Mobile
ADDITIONAL NODE PACKAGES- Desktop
Though, some ptero hosts can have different Startup like this:
![STARTUP (2) - Mobile]()
STARTUP (2) - Mobile
STARTUP (2) - Desktop
In here you can see STARTUP COMMAND 1
and STARTUP COMMAND 2 (OPTIONAL)
, as it says you can have a command which you normally put in a terminal, but it is limited to only 2. So here we set STARTUP COMMAND 1
as npm install aoi.js
and STARTUP COMMAND 2 (OPTIONAL)
as node .
respectively.
Step 4. Create a file called index.js
which we will use as the bot's main file and put this (setup) code.
const { AoiClient } = require("aoi.js");
const client = new AoiClient({
token: "Discord Bot Token",
prefix: "Discord Bot Prefix",
intents: ["MessageContent", "Guilds", "GuildMessages"],
events: ["onMessage", "onInteractionCreate"],
database: {
type: "aoi.db",
db: require("@akarui/aoi.db"),
dbType: "KeyValue",
tables: ["main"],
securityKey: "a-32-characters-long-string-here",
}
});
// Ping Command
client.command({
name: "ping",
code: `Pong! $pingms`
});
You need to replace TOKEN
AND PREFIX
with actual bot token and prefix respectively.
Token would mean a long chain of password which you get from the dev portal.
Prefix means a symbol (or a letter or any word) which comes before a command to indicate the bot that its a command it needs to execute. Though nowadays slash commands serves as a successor to the legacy prefix based commands, but we can still use prefix based commands (For learning how to use slash commands and interaction, you can visit this guide written by dodogames).
As you must have heard, sharing your bot token to someone you don't know or to public can lead to bad consequences for your bot as Bot tokens are really sensitive, If anybody gets your bot token, that person can use your bot for spamming and other stuffs which could break TOS of discord and even do illegal stuffs. So you should take necessary precautions to never reveal your token. Well in a normal ptero server, they use docker to ensure your data/bot token never gets revealed but you should always check your host privacy policies.
We use client
to define your bot here in AoiClient. And it can be changed as well to whatever you like e.g, client
to myfavouritebot
. But doing so, you may need to change how you define your bot in the upcoming time.
We use intents
to define the things the bot can do, use and read in a simple sense. At the moment MessageContent
is a privilege intent so you need to enable that intent
on the developer portal as well. While we use the events
for a particular event, for listening (which means in a sense to retrieve data) when a user joins or leaves a server, what message you have written, etc.
The above setup contains a simple command for you to get the idea on how to create a command.
With this you have finally setup your bot.
Step 5. Now start your server.
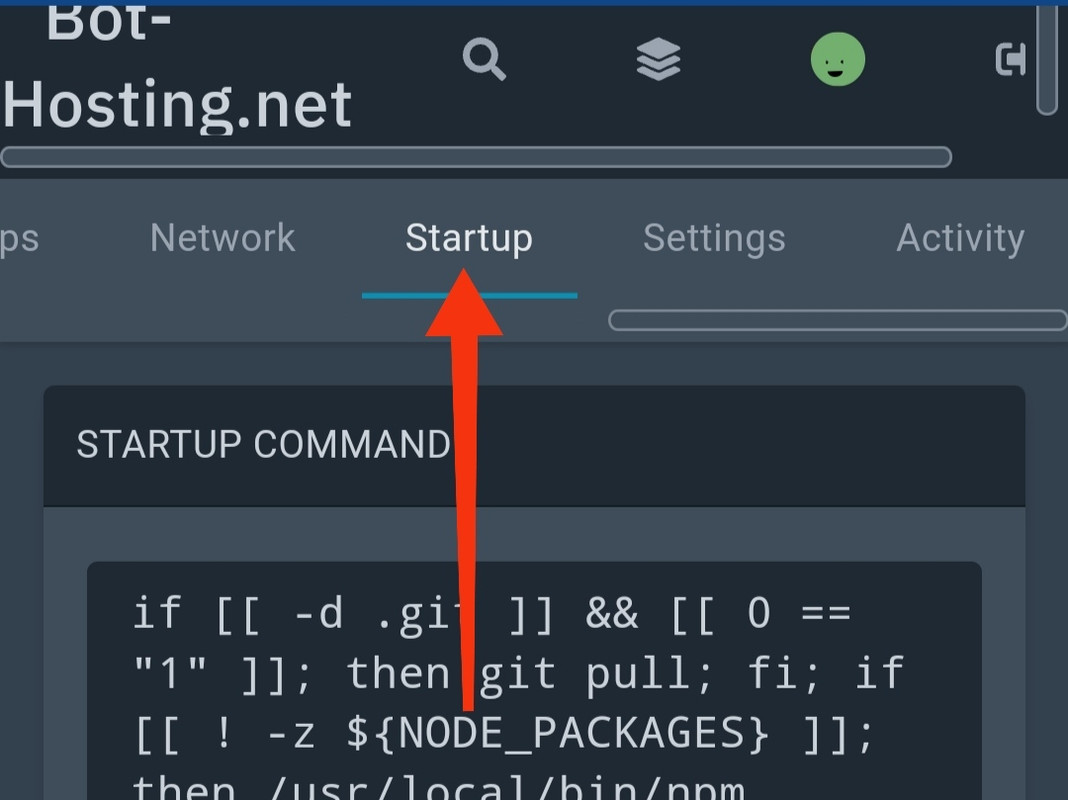
START - Mobile
START - Desktop
Step 6. Hurray! Your bot is ready to use. It should be online if nothing happens.
Now test the command ping
if it works.
How to make a command?
As you can see in the index.js above, I have put a simple command there, we can see that client.command
where the client
can change if you defined your bot with something else other than client
, is used to define a command for the bot. This is actually necessary and it must start and enclose with ({
and })
Now you can see we use a property called name:
which has a string (here its "ping"
) and in this string we define the command name. (We also put a comma ,
in order to accumulate the properties below it.)
Now there is another property called code:
and this is the most important thing in a command. This is where you define the code of the command and it must start and enclose with grave symbol
, in it we see something called $ping
, this is a function, the $ping
function returns the ping of the bot. We have like 640+ functions like this functions which can be used to create a lot of commands, you can check them in the docs.
REMEMBER: AOI.JS READS FROM BOTTOM TO TOP FOR COMMANDS
There are other properties like alias
and events commands. But we won't cover them in this guide, you can always go to the docs for learning about the command options.
How to make my commands organized and actually fitting all of my bot commands in my bot main file won't make it laggy? I want to know more about this so-called Command Handler?
Yeah, it will make your commands unorganized and trying to fit all the commands of your bot in your bot main file will make it extremely laggy and slow.
As a solution to this, we have introduced: Command Handler
It let's your bot store your commands in a seperate folder, with each of your command having its own dedicated folder.
Let's get started on how to make it work.
We add this line in the index.js at the below:
client.loadCommands("./commands/", true)
Here the first value, ./commands/
is the path where your bot will store the commands.
Second value true
, it denotes whether it should log which-which commands have loaded. Here it is set true
so it will log but setting it false
it will stop logging.
We create a folder called commands
(depends upon the path you selected) and you may even create sub-folders inside it.
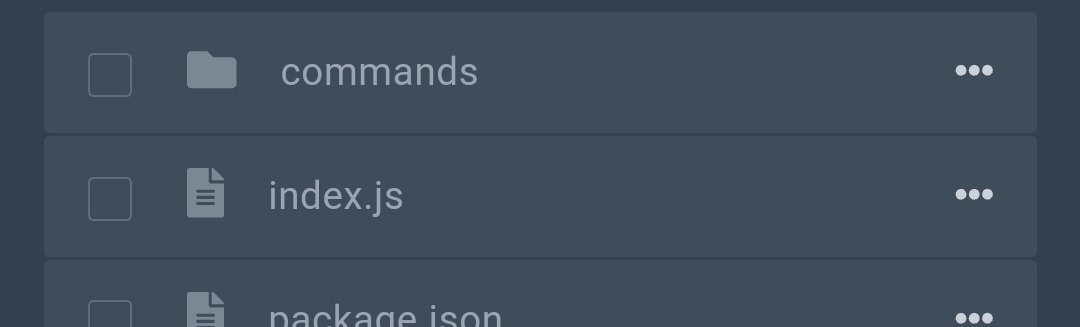
FOLDER
Now, we create a file inside the commands
(or any other folder you selected as path) folder or inside its sub-folders called <command>.js
where we replace <command>
with a actual command name but make sure it has the extension js
, e.g, help.js
.
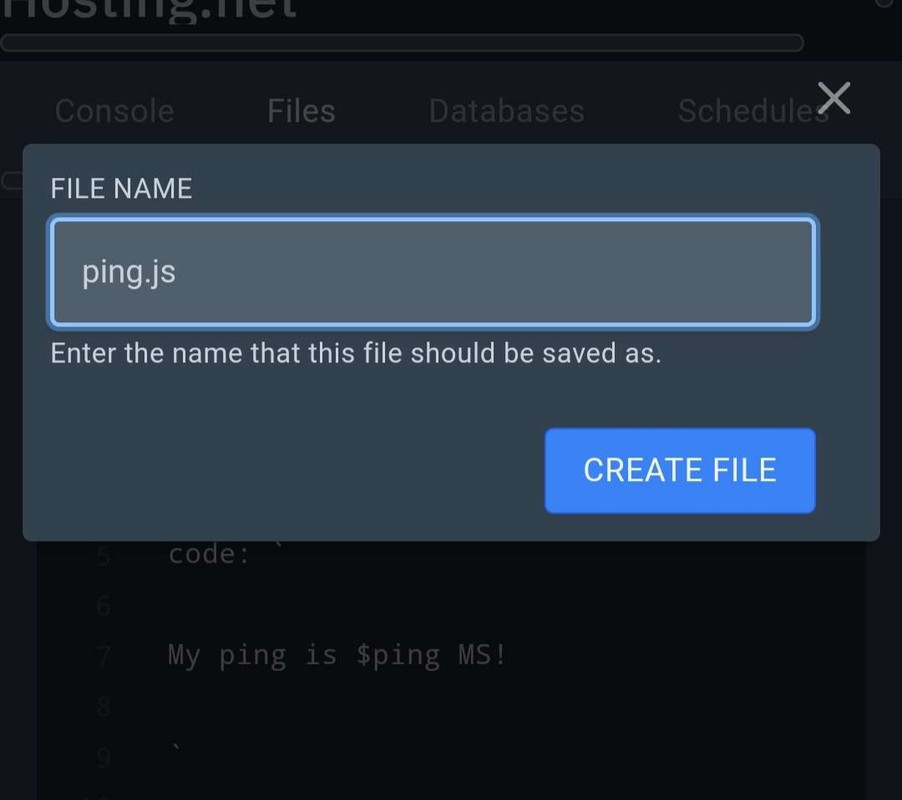
CH EXAMPLE- Mobile
CH EXAMPLE- Desktop
Remember you must create command files inside commands
folder or else command handler won't work.
Now, we need to change our method of creating commands, it mostly remains unchanged except 2-3 things.
module.exports = {
name: `ping`,
code: `
My ping is $ping MS!
`
}
We need to use module.exports = {...}
now instead of client.command({...})
.
Remember you must type the code in a file created within the folder commands
(can change depending upon the path you selected) or its sub-folders.
Note, you can create a group of commands in a single file using command handler which makes it easy to use, but for it you need to change module.exports = {...}
to module.exports = [{...}]
, now we can create as many as we need nested commands. e.g,
module.exports = [{
name: `ping`,
code: `
My ping is $ping MS!
`
}, {
name: "pong",
code: `
My pong is š
`
}]
I always recommend you to use Command Handler instead of making commands in your bot main file.
Alright, I see I can create commands, how do I use this variable
and status
thingy?
Variables
Variables are like some kind of containers where we store things, in this case values
. We store values for later use.
In order to make variables, we use this code, put it in index.js:
client.variables({
blacklisted: false,
money: 0,
title: "none",
});
We have a variable name (e.g, "title") and a value (e.g "none").
Variables value can be anything, a string, integer, boleen, object.
We add a variable name, put a :
, add the value depending on the type and lastly put a ,
(if there is an another variable below it) .
Status
Status are like umm.... How should I say... They display some kind of message in their profile like this:
STATUS
Now, for making a status we code in index.js the following:
client.status({
name: string,
type: string,
time: number,
URL?: string,
afk?: boolean
});
There are basically six types of status you can use:
PLAYING
WATCHING
STREAMING
LISTENING
COMPETING
CUSTOM
The six types are self-explaining. Playing denotes a playing status, watching a watching status and so on. While custom means that there won't anything before your status which for other type will, e.g, for PLAYING, a playing text will be written before your bot status.
We need a name
which means the status text or in other words your status content.
The type
is basically those what I said above.
time
represents the time for which the current status will remain before switching to the second status (if any) or loop the current status.
url
is basically needed when you have type STREAMING
and as it says, you need a URL
to put here while afk
is what it says, if you want the bot to be afk. Both of them are optional and can be removed.
e.g,
client.status({
name: "Example Text one!",
type: "PLAYING",
time: 12,
});
You can also set a bot presence, which I mean if you want the bot to be online
or dnd
or invisible
.
There are four types of presences:
online
idle
dnd
invisible
You can set the presence by adding the status:
property. e.g,
client.status({
name: "I am a busy bot!",
type: "CUSTOM",
status: "dnd",
time: 12,
});
With this, we can say that the bot is fully functional and can be used.
You may check the original docs for a lot of information. In fact, I recommend you to read the docs
FAQ
Are this everything aoi.js has?
NO,
Whatever I have explained above are just a tip of a iceberg. Aoi.js has lot, lot and lot of stuffs.
You can always read their docs for more information.
Is aoi.js by any way affiliated/associated to discord?
No, Aoi.js is not affiliated or associated with discord in any way. We only provide a simple wrapper for their API to help developer to make their bot easily.
I have seen other bot-creating language like this, are they related with aoi.js?
No, They are not related with aoi.js. We may have taken inspiration from them or vice versa but that's probably the only reason for similarily between us.
What is this DBD.js? Should I use it?
DBD.js is like a way outdated version of aoi.js. You can also think of aoi.js as the successor to DBD.js.
No, you shouldn't use it. As it contains a lot of bugs and issues plus it is discontinued so we won't provide support for it in any possible way.
What about I don't understand something? How will I ask?
We have a discord server where you can ask us if you don't understand, we will help you!
For now bye I hope you understood what I said if you don't, please come to our server we will help you.
I thank you for using Aoi.js and reading this guide.
A special thanks and credit goes to dodogames for his contribution in this guide. Without him, this guide wouldn't have been completed. He has provided all the desktop view images you have seen above.
A big thanks also goes to each and every Akarui staffs and developers for their utmost dedication in maintaining the server/helping the users and in developing aoi.js and its extensions respectively
If you found any error, typo, missing stuffs, outdated stuffs, etc., please do message me in this discussion.
Related guides!
Migrating from BDFD to Aoi.js
Getting started with slash commands! by dodogames
Statics
Total Character Count^: 16160 characters
Total Word Count^: 2492 words
Published on: 20/02/2024
Last Updated on: 20/02/2024
^ Includes the markdown text count as well
Calculated using wordcounter.net